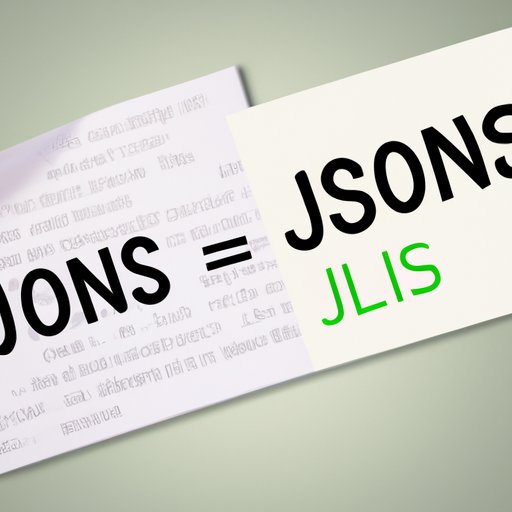
How to Open JSON Files: A Comprehensive Guide for Beginners
If you’re working with JSON data, it’s important to know how to open and read JSON files. JSON stands for JavaScript Object Notation and is a lightweight data interchange format that is easy to read and write. JSON files are commonly used to store and exchange data over the internet, making them an essential component of many modern software applications.
In this article, we will provide a comprehensive guide on how to open JSON files. We will cover everything from identifying JSON files, checking syntax, to a step-by-step guide for opening and reading JSON files in different operating systems and programming languages. By the end of this article, you will have a thorough understanding of how to work with JSON files and be able to extract information using simple commands.
A Comprehensive Guide to Opening a JSON File: Tips and Tricks for Beginners
Before we dive into the specifics of opening and reading JSON files, let’s first talk about what you need to work with JSON files. The good news is that you don’t need any special software or tools to open JSON files. All you need is a plain text editor or any web browser capable of opening JSON files.
Once you have your text editor or web browser ready, you can start by identifying the JSON file you want to work with. JSON files commonly have a .json file extension and are usually structured as objects with key-value pairs. However, it’s important to check the syntax of the JSON file to avoid errors when reading and manipulating the data.
Common syntax errors in JSON files include missing commas, incorrect use of brackets and braces, and duplicate keys. If you encounter any syntax errors, fixing them is often a matter of looking for typos or adding missing characters. You can also use an online JSON validator to check the syntax of your JSON file.
Step-by-Step Guide to Open and Read a JSON File in Different Programming Languages
To open and read JSON files in different programming languages, you need to understand the basic syntax and commands first. Here are some examples of how to open and read a JSON file using popular programming languages:
Python:
Python provides a simple and efficient way to work with JSON data using its built-in library called json. Here’s an example of how to open and read a JSON file in Python:
import json
with open('data.json') as f:
data = json.load(f)
print(data)
JavaScript:
JavaScript is the language behind JSON, so it’s no surprise that it handles JSON data natively. Here’s an example of how to open and read a JSON file in JavaScript:
fetch('data.json')
.then(response => response.json())
.then(data => console.log(data));
Java:
Java has a built-in library called Gson (Google’s JSON library for Java) for reading and writing JSON in Java. Here’s an example of how to open and read a JSON file in Java:
import com.google.gson.Gson;
import java.io.FileReader;
import java.io.IOException;
public class ReadJSON {
public static void main(String[] args) {
Gson gson = new Gson();
try (FileReader reader = new FileReader("data.json")) {
// Convert JSON to Java object
Object obj = gson.fromJson(reader, Object.class);
System.out.println(gson.toJson(obj));
} catch (IOException e) {
e.printStackTrace();
}
}
}
These are just a few examples of how to open and read JSON files in different programming languages. There are many libraries and tools available for working with JSON data in different programming languages. You can also find examples of code snippets for different programming languages online to help you get started.
How to Open a JSON File: Explained with Interactive Examples for Different Operating Systems
Once you know how to open and read a JSON file in different programming languages, you need to understand how to do the same in different operating systems. Here’s an overview of how to open a JSON file on different operating systems:
Windows:
To open and read a JSON file on Windows, you can use any text editor like Notepad, Wordpad, or any other free software available online. Here are the steps to open a JSON file on Windows:
- Locate the JSON file you want to open
- Right-click on the JSON file and select “Open With” from the context menu
- Select the text editor of your choice from the list of available applications
- The JSON file will now open in the selected text editor and you can start working with the data
Mac:
Mac users can also use a text editor or web browser to open and read JSON files. The steps to open a JSON file on a Mac are as follows:
- Locate the JSON file you want to open
- Right-click on the JSON file and select “Open With” from the context menu
- Select a text editor or web browser from the list of available applications
- The JSON file will now open in the selected program or web browser, and you can start working with the data
Linux:
Linux users have several options for opening and reading JSON files. Like Windows and Mac OS, Linux users can also use a text editor or web browser to open and read JSON files. The steps to open a JSON file on a Linux system are as follows:
- Open the terminal
- Use the cd command to navigate to the directory where the JSON file is stored
- Type “nano filename.json” to open the JSON file in the Nano text editor
- You can now start working with the data in the JSON file
Simplifying the Process of Opening JSON Files on Windows, Mac, and Linux Systems
If you’re looking to simplify the process of opening JSON files, there are several tools and software that can help. Here’s an overview of some of the tools and software available to make working with JSON files easier:
Text Editors:
Text editors like Sublime Text, Atom, and Notepad++ support syntax highlighting for JSON files, which makes it easier to read and identify errors in the syntax. In addition, many text editors offer plugins that can simplify the process of working with JSON data.
JSON Viewers:
JSON viewers are dedicated tools designed for viewing and exploring JSON data. These tools offer a user-friendly interface that makes it easy to read and manipulate JSON data. There are many free and paid options available online.
Web Browser Plugins:
Web browser plugins like JSONView and JSON Formatter & Validator can simplify the process of opening and reading JSON files in web browsers. With these plugins installed, you can easily view and validate JSON data directly in your web browser.
When choosing a tool or software to work with JSON data, it’s important to consider your specific needs and preferences. While some tools may be more user-friendly, others may offer advanced features that are essential for more complex JSON data manipulations.
Unlocking the Secrets of JSON: A Guide to Opening and Parsing JSON Files in Any System
Opening and parsing JSON files is essential for working with JSON data. Parsing refers to the process of analyzing a piece of text and identifying its syntax structure. Here’s a step-by-step guide to parsing JSON files in different programming languages:
Python:
Python’s built-in json library provides functions for parsing JSON data. Here’s an example of how to parse a JSON file in Python:
import json
with open('data.json') as f:
data = json.load(f)
# Parse the JSON data
parsed_data = json.loads(json.dumps(data))
print(parsed_data)
JavaScript:
JavaScript’s JSON.parse() method is used to parse JSON data. Here’s an example of how to parse a JSON file in JavaScript:
fetch('data.json')
.then(response => response.json())
.then(data => {
// Parse the JSON data
const parsed_data = JSON.parse(JSON.stringify(data));
console.log(parsed_data);
});
Java:
Gson provides methods for parsing JSON data in Java. Here’s an example of how to parse a JSON file in Java:
import com.google.gson.Gson;
import java.io.FileReader;
import java.io.IOException;
public class ParseJSON {
public static void main(String[] args) {
Gson gson = new Gson();
try (FileReader reader = new FileReader("data.json")) {
// Parse JSON to Java object
Object obj = gson.fromJson(reader, Object.class);
// Convert the Java object back to JSON
String json = gson.toJson(obj);
System.out.println(json);
} catch (IOException e) {
e.printStackTrace();
}
}
}
These are just a few examples of how to parse JSON files in different programming languages. Once you have parsed a JSON file, you can start manipulating and working with the data in a more meaningful way.
From File to Data: How to Open and Extract Information from JSON Files with Simple Commands
While opening and reading JSON files is essential, extracting specific information from JSON files is even more important. Here are some examples of how to extract specific data fields from a JSON file:
Python:
Python provides a simple way to extract specific data fields from JSON data using the dictionary syntax. Here’s an example of how to extract specific data fields from a JSON file in Python:
import json
with open('data.json') as f:
data = json.load(f)
# Extract fields from JSON data
name = data['name']
age = data['age']
print(name, age)
JavaScript:
JavaScript provides a way to use the dot notation or bracket notation to extract specific data fields from JSON objects. Here’s an example of how to extract specific data fields from a JSON file in JavaScript:
fetch('data.json')
.then(response => response.json())
.then(data => {
// Extract fields from JSON data
const name = data.name;
const age = data.age;
console.log(name, age);
});
Java:
Gson provides methods for extracting specific data fields from JSON data in Java. Here’s an example of how to extract specific data fields from a JSON file in Java:
import com.google.gson.Gson;
import java.io.FileReader;
import java.io.IOException;
public class ExtractJSON {
public static void main(String[] args) {
Gson gson = new Gson();
try (FileReader reader = new FileReader("data.json")) {
// Extract fields from JSON data
Data data = gson.fromJson(reader, Data.class);
String name = data.name;
int age = data.age;
System.out.println(name + " " + age);
} catch (IOException e) {
e.printStackTrace();
}
}
class Data {
String name;
int age;
}
}
With these simple commands, you can extract specific information from a JSON file and work with it in different ways.
Conclusion
In this article, we’ve covered everything you need to know about opening and reading JSON files. We started by explaining what a JSON file is, why it’s important, and provided tips and tricks for identifying JSON files and checking their syntax. We then provided step-by-step guides on how to open and read JSON files in different programming languages and operating systems. We also discussed tools and software that can simplify the process of working with JSON files, unlocking the secrets of JSON, and extracting information from JSON files with simple commands.
With this knowledge, you can start working with JSON files and extract valuable information from them in any programming language or system. We hope this guide has been helpful in getting you started on your journey to becoming a JSON expert.