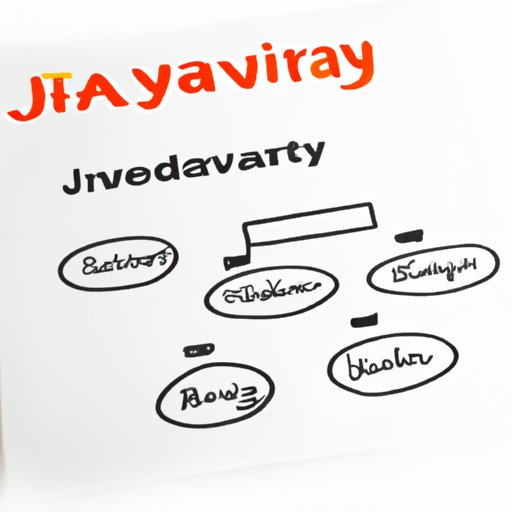
Introduction
If you are new to Java programming, you might encounter the challenge of initializing an array. An array is a collection of elements of the same type that are stored in contiguous memory locations. Initializing an array means giving values to its elements at the time of creation. There are several ways to initialize an array in Java, each with its own syntax, usage, and advantages. In this article, we will explore these methods in detail to help you choose the best approach for your specific needs.
Using the curly brace syntax to initialize values in an array
The curly brace syntax is the simplest and most straightforward way to initialize an array in Java. It entails enclosing the values in curly braces and separating them with commas, as shown in this example:
int[] numbers = {1, 2, 3, 4};
This creates an integer array named numbers
with four elements, each initialized to the respective value. The curly brace syntax is most useful for small, fixed-size arrays with known values.
Syntax and usage
The syntax for using the curly brace syntax to initialize an array is:
datatype[] arrayName = {value1, value2, ..., valueN};
Where:
datatype
is the data type of the elements in the array.arrayName
is the name of the array.value1, value2, ..., valueN
are the values to be assigned to the elements of the array.
Examples
The curly brace syntax can be used to initialize arrays of any data type, including:
- Integers
- Characters
- Strings
- Booleans
- Arrays of any other type
Here are some examples:
//Initializing integer array
int[] numbers = {1, 2, 3, 4};
// Initializing character array
char[] letters = {'a', 'b', 'c', 'd'};
// Initializing string array
String[] fruits = {"apple", "banana", "orange"};
// Initializing boolean array
boolean[] flags = {true, false, true, false};
// Initializing array of arrays
int[][] matrix = {{1, 2}, {3, 4}};
Pros and cons
The curly brace syntax is the simplest and most concise way to initialize an array in Java. It is especially useful for small arrays with a fixed number of values. The downside is that it can be cumbersome for large arrays or arrays with complex values. Additionally, it only works at the time of declaration, so you cannot use it to reinitialize an array later on.
Using a for loop to populate elements in an array with values
A for loop is another common way to initialize an array in Java. In this approach, you declare the array and then use a for loop to loop through its elements and assign them values based on a logic or algorithm. The for loop approach is useful when you need to populate the array with values that cannot be easily hardcoded.
Syntax and usage
The syntax for using a for loop to initialize an array is:
datatype[] arrayName = new datatype[arraySize];
for(int i=0; i<arraySize; i++) {
arrayName[i] = value;
}
Where:
datatype
is the data type of the elements in the array.arrayName
is the name of the array.arraySize
is the size of the array.i
is a counter variable that loops through the elements of the array.value
is the expression that is evaluated and assigned to each element of the array.
Examples
Here are some examples of using a for loop to initialize an array:
// Initializing an integer array
int[] numbers = new int[4];
for(int i=0; i<4; i++) {
numbers[i] = i + 1;
}
// Initializing a string array
String[] words = new String[3];
for(int i=0; i<3; i++) {
words[i] = "word " + (i+1);
}
Pros and cons
The for loop approach is flexible and can handle any type of array initialization logic, including randomly generated or user-input values. It is also useful for dynamically sized arrays, where the size is not known at the time of declaration. However, it can be more verbose and harder to read than the curly brace syntax. It can also be slower for large arrays or complex initialization logic.
Using the Java Arrays.fill() method to initialize all elements in an array with a single value
The Java Arrays class provides several utility methods for working with arrays, including the fill()
method. This method takes an array and a single value, and then sets all the elements of the array to that value. The fill()
method is useful when you need to initialize an array with a default value or reset an array to a known state.
Syntax and usage
The syntax for using the Arrays.fill() method to initialize an array is:
Arrays.fill(arrayName, value);
Where:
arrayName
is the name of the array.value
is the value to be assigned to all elements of the array.
Examples
Here are some examples of using the Arrays.fill() method to initialize an array:
// Initializing an integer array
int[] numbers = new int[4];
Arrays.fill(numbers, 0);
// Initializing a string array
String[] words = new String[3];
Arrays.fill(words, "java");
Pros and cons
The fill()
method is a simple and efficient way to initialize an array with a single value. It is especially useful for setting default values or resetting the array between uses. However, it is not useful for arrays with complex values or varying values. Additionally, it modifies the array in-place, so be careful when using it with arrays that are already in use.
Using the System.arraycopy() method to copy a range of values into an array
The System class provides several utility methods for working with the system and memory, including the arraycopy()
method. The arraycopy()
method takes the source array, the starting index, the destination array, the starting index, and the number of elements to copy, and then copies the specified range of the source array into the destination array. The arraycopy()
method is useful when you need to copy a range of values from one array to another or initialize an array with values from another array.
Syntax and usage
The syntax for using the System.arraycopy() method to initialize an array is:
System.arraycopy(sourceArray, srcPosition, destArray, destPosition, length);
Where:
sourceArray
is the source array whose elements will be copied.srcPosition
is the starting index of the range of elements to copy from the source array.destArray
is the destination array where the copied elements will be placed.destPosition
is the starting index in the destination array where the copied elements will be placed.length
is the number of elements to copy.
Examples
Here are some examples of using the System.arraycopy() method to initialize an array:
// Initializing an integer array using values from another array
int[] sourceArray = {1, 2, 3, 4};
int[] targetArray = new int[sourceArray.length];
System.arraycopy(sourceArray, 0, targetArray, 0, sourceArray.length);
// Initializing a string array using values from a portion of another array
String[] sourceWords = {"apple", "banana", "cherry", "date"};
String[] targetWords = new String[2];
System.arraycopy(sourceWords, 1, targetWords, 0, 2);
Pros and cons
The arraycopy()
method is useful for copying a range of values from one array to another or initializing an array with part of another array. It is efficient and can save memory by avoiding unnecessary copying of unused elements. However, it requires understanding of the position and length parameters, which can be confusing for some users. It is also not useful for initializing arrays with a single default value or complex values.
Using the Arrays.copyOf() method to create a new array and populate it with existing values
The Arrays class also provides the copyOf()
method, which can be used to create a new array and copy a portion of an existing array or fill it with a default value. The copyOf()
method is useful when you need to create a new array based on an existing one or extract a range of values from an array.
Syntax and usage
The syntax for using the Arrays.copyOf() method to initialize an array is:
datatype[] newArray = Arrays.copyOf(sourceArray, length);
Where:
datatype
is the data type of the elements in the array.newArray
is the name of the new array to be created.sourceArray
is the source array whose elements will be copied.length
is the number of elements to copy.
Examples
Here are some examples of using the Arrays.copyOf() method to initialize an array:
// Initializing a new integer array with the values from an existing integer array
int[] oldArray = {1, 2, 3, 4};
int[] newArray = Arrays.copyOf(oldArray, oldArray.length);
// Initializing a new string array with default values
String[] words = new String[3];
String[] newWords = Arrays.copyOf(words, words.length);
Arrays.fill(newWords, "java");
Pros and cons
The copyOf()
method is useful for creating a new array based on an existing one or copying a portion of an array. It is efficient and can save memory by copying only the necessary elements. However, like the arraycopy()
method, it requires understanding of the length parameter, which can be confusing for some users. Additionally, it is not useful for initializing arrays with complex values or dynamic values.
Using the Java stream API to initialize an array with a sequence of values
The Java stream API provides a concise and powerful way to work with collections, including arrays. You can use the stream()
, toArray()
, and other stream methods to create a stream of elements and then convert them into an array. The stream API approach is useful for initializing arrays with a sequence of values or applying a transformation to the values before storing them in an array.
Syntax and usage
The syntax for using the Java stream API to initialize an array is:
datatype[] newArray = streamOfElements.toArray(datatype[]::new);
Where:
datatype
is the data type of the elements in the array.newArray
is the name of the new array to be created.streamOfElements
is a stream of elements that will be converted into an array.
Examples
Here are some examples of using the Java Stream API to initialize an array:
// Initializing an integer array with a sequence of numbers
int[] numbers = IntStream.range(1, 11).toArray();
// Initializing a string array with values transformed from another array
String[] words = {"apple", "banana", "cherry"};
String[] newWords = Arrays.stream(words).map(w -> w.substring(0, 1)).toArray(String[]::new);
Pros and cons
The Java stream API approach is concise and powerful, enabling you to initialize an array with a sequence of values or apply a transformation to the values before storing them in an array. It is especially useful when working with complex or large collections of data. However, it requires familiarity with the stream methods and syntax, which can be challenging for some users. It can also be slower than other approaches due to the overhead of creating and working with a stream.
Using a static initializer to initialize values in an array at the time of declaration
A static initializer is a block of code that is executed when a class is loaded into memory. You can use a static initializer to initialize values in an array at the time of declaration. This approach is useful when you need to initialize an array with complex or hard-to-calculate values and don’t want to repeat the calculation each time the array is used.
Syntax and usage
The syntax for using a static initializer to initialize values in an array is:
datatype[] arrayName = {
expression1,
expression2,
expression3,
...