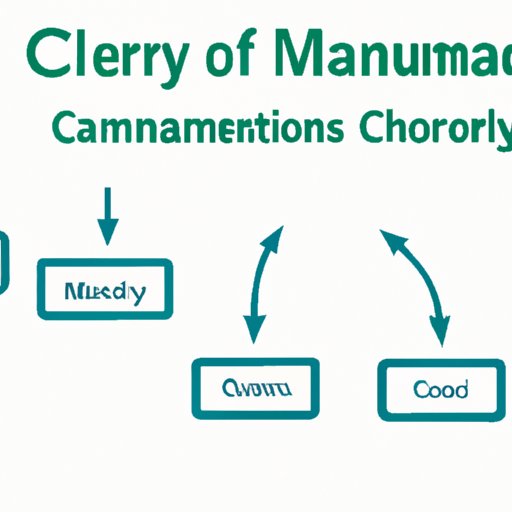
I. Introduction
If you are programming in C, you will inevitably deal with memory management. One crucial aspect of memory management is freeing an array when you are done using it. Failing to do so can lead to memory leaks and impact the overall performance of your program. Therefore, in this article, we will provide a thorough guide on the basic process of freeing an array in C so that you can optimize the performance of your program.
II. Basic Process of Freeing an Array in C
In C, an array is allocated using functions like malloc()
or calloc()
. To free an array, you need to call the function free()
. Here is the syntax you would use:
free(pointer_variable);
Where pointer_variable
is a pointer to the memory you want to free.
It is essential to note that you should only attempt to free memory that has already been allocated. Otherwise, it could cause your program to crash.
III. Tips for Troubleshooting Common Issues
When dealing with memory management, you will often face bugs related to memory leaks and segmentation faults. Here are some tips for troubleshooting these issues:
A. Suggestions for debugging memory leaks:
- Use a tool like Valgrind or AddressSanitizer to detect memory leaks
- Track your allocated memory by keeping a count of how many times you allocate and deallocate it
- Use calloc() instead of malloc() as it initializes the allocated memory to 0
B. Tips for resolving other common problems related to freeing an array in C:
- Make sure that you are not freeing memory that has already been freed
- Avoid casting the result of malloc() or calloc() as it can mask casting mistakes
- Initialize pointers to NULL after freeing memory to prevent segmentation faults
IV. Best Practices for Managing Memory in C
Managing memory effectively is crucial to optimizing the performance of your program. Here are some best practices for memory management in C:
A. Tips for optimizing performance:
- Allocate only the memory you need
- Minimize fragmentation by freeing memory as soon as you are done using it
- Use a memory pool to avoid the overhead of allocating and deallocating memory repeatedly
B. Ways to avoid memory errors:
- Avoid accessing memory after it has been freed
- Avoid using uninitialized memory as it can contain unpredictable values
- Initialize all variables when you declare them
C. Ways to create efficient code:
- Use data structures that are designed to minimize memory usage, such as bitfields and hash tables
- Avoid passing large data structures as arguments to functions
- Use const pointers to increase the security of your code and potentially optimize it
V. Advanced Techniques and Strategies
A. Garbage collection and how to use it:
Garbage collection is an automatic memory management technique that frees memory that is no longer needed by the program. In C, you can use a garbage collector library like Boehm-Demers-Weiser’s GC to implement garbage collection.
B. Discussion on low-level memory manipulation:
Low-level memory manipulation is the process of manipulating individual bits of data in memory to create more efficient code. Techniques include bit-twiddling and bit-shifting.
C. Other advanced coding strategies:
- Use manual memory management techniques like reference counting to optimize performance
- Create custom allocators to improve performance
VI. Examples and Code Snippets
To illustrate how to free arrays in C, here are some real-world examples and code snippets:
int* array = (int*) calloc(10, sizeof(int));
// do something with array
free(array);
The code above uses calloc()
to allocate an array of 10 integers and then frees it using free()
once it is no longer needed.
VII. Conclusion
By now, you should understand the importance of freeing an array in C and have a good grasp of the basic process of doing so. Remember to follow best practices for memory management to optimize the performance of your program, and troubleshoot common issues like memory leaks and segmentation faults using the tips discussed in this article.