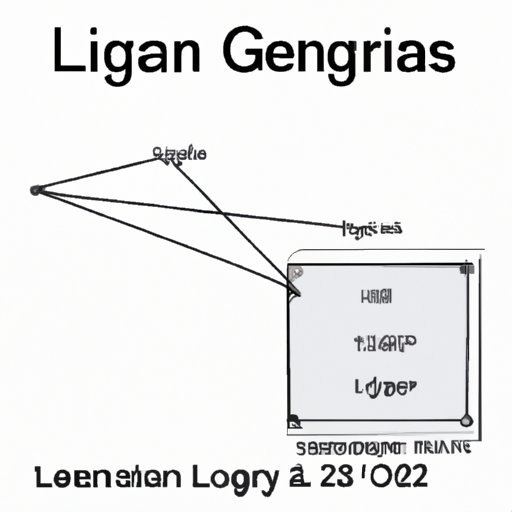
Introduction
Understanding eigenvectors is crucial for various fields, including data analysis, physics, and computer science. This article will provide a step-by-step guide on how to find eigenvectors, explain their importance in different fields, compare different methods for finding them, and offer real-world examples.
Step-by-step guide on how to find eigenvectors
An eigenvector is a vector whose direction remains unchanged after a linear transformation. Finding eigenvectors involves solving the characteristic equation of a matrix. The formula for calculating eigenvectors is:
Av = λv
Where A is the matrix, λ is the eigenvalue, and v is the eigenvector. To solve for v, we can rearrange the formula:
(A – λI)v = 0
Where I is the identity matrix. This equation can be solved using Gaussian elimination or any other method for solving linear equations. The resulting solution for v will be the eigenvector.
Let’s take the matrix A = [1 3; 3 1] as an example:
First, we will solve for the eigenvalues:
| A – λI | = 0
| 1-λ 3 | = (1-λ)(1-λ) – 9 = 0
| 3 1-λ |
Solving this equation, we get λ1 = 4 and λ2 = -2.
Next, we will solve for the eigenvectors:
For λ1 = 4:
(A – λ1I)v =
| -3 3 | | x | | 0 |
| 3 -3 | * | y | = | 0 |
Solving this equation, we get v1 = [1 1].
For λ2 = -2:
(A – λ2I)v =
| 3 3 | | x | | 0 |
| 3 3 | * | y | = | 0 |
Solving this equation, we get v2 = [-1 1].
Therefore, the eigenvectors for A are v1 = [1 1] and v2 = [-1 1].
Importance of eigenvectors in various fields
Eigenvectors play a significant role in data analysis and physics. In data analysis, eigenvectors are used for principal component analysis (PCA), which is a technique for reducing the dimensionality of data while retaining its most significant features. In physics, eigenvectors are used to represent the state of a physical system.
For example, in quantum mechanics, eigenvectors represent the possible states of a system, and the corresponding eigenvalues represent the energy of those states. In fluid dynamics, eigenvectors of a matrix can describe the directional characteristics of fluid flow.
Real-world applications of eigenvectors include image and speech recognition, signal processing, financial modeling, and network analysis.
Comparison of different methods for finding eigenvectors
There are several methods for finding eigenvectors, including the power method, QR algorithm, and Jordan decomposition.
The power method is a numerical algorithm that estimates the eigenvector corresponding to the largest eigenvalue of a matrix. It works by repeatedly applying A to a starting vector until it converges to the eigenvector. The power method is fast and efficient but can only find the eigenvector corresponding to the largest eigenvalue.
The QR algorithm is a numerical algorithm that iteratively transforms a matrix into upper triangular form by applying orthogonal matrices. It works by decomposing an input matrix into orthogonal and upper-triangular factors and then iteratively applying the same process until convergence. The QR algorithm can find all the eigenvectors and eigenvalues of a matrix but can be computationally expensive for larger matrices.
Jordan decomposition involves decomposing a matrix A into two matrices J and P, where J is a related diagonal matrix and P is the invertible matrix of eigenvectors. Jordan decomposition can be used to find all the eigenvectors of a matrix, but it is more complex than the other methods and can be numerically unstable in some cases.
Real-world examples of where eigenvectors are used
Eigenvectors are used extensively in various fields, including computer science and network analysis. In computer science, eigenvectors are used in Google’s PageRank algorithm, which determines the importance of web pages based on their links to other pages. The eigenvector corresponding to the largest eigenvalue of the link matrix is used to rank the pages.
In network analysis, eigenvectors are used to identify the most influential nodes in a network. The eigenvector corresponding to the largest eigenvalue of the adjacency matrix is used to identify the most influential nodes.
Common misconceptions or mistakes people make when trying to find eigenvectors
One common mistake people make when trying to find eigenvectors is to mix up the order of the eigenvalues and eigenvectors. It is essential to match each eigenvalue to its corresponding eigenvector.
Another common mistake is to assume that a matrix has only one eigenvector or eigenvalue. This assumption is incorrect, as most matrices have multiple eigenvectors and eigenvalues.
Finally, it can be tempting to use software packages to find eigenvectors without understanding the underlying concepts. However, this approach can lead to errors and does not promote a deeper understanding of the topic.
Programming examples and code snippets
Implementing eigenvector calculations can be challenging, but there are several libraries available in programming languages such as Python and MATLAB that simplify the process. Here is an example code for finding eigenvectors using Python’s NumPy library:
import numpy as np
A = np.array([[1, 3], [3, 1]])
eigenvalues, eigenvectors = np.linalg.eig(A)
print(“Eigenvalues:”, eigenvalues)
print(“Eigenvectors:”, eigenvectors)
Similarly, here is an example code for finding eigenvectors using MATLAB:
A = [1 3; 3 1];
[ev, lambda] = eig(A);
disp(“Eigenvalues:”);
disp(lambda);
disp(“Eigenvectors:”);
disp(ev);
Historical context on the development of eigenvectors
The concept of eigenvectors was first introduced by British physicist William Rowan Hamilton in the mid-19th century. However, the modern formulation of eigenvectors stems from the work of German mathematician David Hilbert and his contemporaries in the late 19th and early 20th centuries.
The development of eigenvectors played a significant role in the evolution of quantum mechanics, where they are used to describe the possible states of a physical system. The use of eigenvectors in physics and other fields has continued to evolve and expand to this day.
Conclusion
Eigenvectors are an essential concept in various fields, including data analysis, physics, and computer science. This article provided a step-by-step guide on how to find eigenvectors, explained their importance in different fields, compared different methods for finding them, and offered real-world examples. It also highlighted common misconceptions and mistakes people make and provided programming examples and code snippets. By understanding the concept of eigenvectors, readers can expand their knowledge and apply it to their own projects and research.