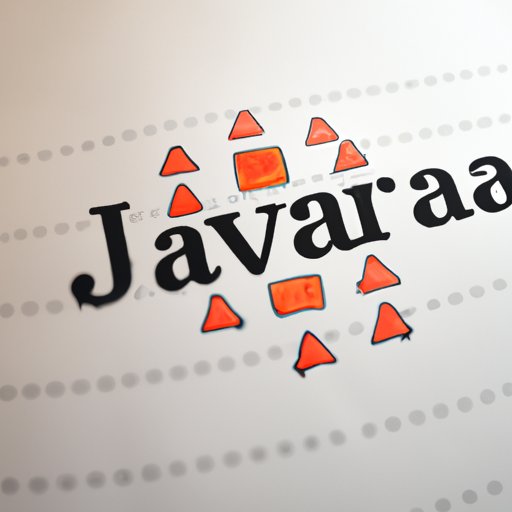
Introduction
Working with arrays is an essential aspect of programming in Java. However, array declaration can be a challenging concept for beginners to grasp. In Java, you need to declare an array before using it, and it involves specifying the type of elements you want to store, as well as the number of elements in the array. This article provides a comprehensive guide on how to declare an array in Java.
A Comprehensive Guide on How to Declare an Array in Java
An array is a data structure that stores a fixed-size collection of elements of the same data type. In Java, arrays are objects, which means they are dynamically created and can be assigned to a variable.
Syntax of Declaring an Array
Declaring an array involves specifying the type of the array, followed by the array name, and the number of elements in square brackets. Here is the general syntax for declaring an array in Java:
datatype[] arrayName = new datatype[length];
Here, datatype
is the data type of the array, arrayName
is the name of the array, and length
is the number of elements in the array.
Examples of Integer, String, and Multi-Dimensional Arrays
Let’s take a look at some examples of array declaration in Java.
Integer Array:
int[] numbers = new int[5];
This code declares an integer array named numbers
that can hold five integer values.
String Array:
String[] myWords = new String[3];
This code declares a String array named myWords
that can hold three String values.
Multi-Dimensional Array:
int[][] myMatrix = new int[3][3];
This code declares a two-dimensional array named myMatrix
that can hold up to nine integer values.
Top 5 Java Methods to Declare Arrays
In Java, there are several ways to declare arrays. Here are the top five methods:
Method 1: Declaring an Array with the “new” Keyword
One of the most common ways of declaring an array in Java is by using the “new” keyword. This method is useful when you don’t know the values to be stored in the array at the time of declaration.
Here is an example of declaring an array using the new keyword:
String[] fruits = new String[3];
This code creates a String array named fruits
with three elements.
Method 2: Short-Hand Syntax
Java provides a shorthand syntax for declaring and initializing an array in a single line of code.
Here is an example of using the shorthand syntax:
int[] numbers = {1, 2, 3, 4, 5};
This code creates an integer array named numbers
with five elements and initializes them with the values 1, 2, 3, 4, and 5.
Method 3: Using the Anonymous Array
Java allows you to create an anonymous array without assigning it a variable. This method is useful when you only need the array temporarily and don’t want to declare a variable for it.
Here is an example of using an anonymous array:
System.out.println("The sum of the values is " + (new int[]{1, 2, 3}[0] + new int[]{1, 2, 3}[1] + new int[]{1, 2, 3}[2]));
This code prints out the sum of the integer values 1, 2, and 3, which are part of an anonymous array.
Method 4: Initializing an Array with Default Values
Java automatically assigns default values to array elements based on the data type. For example, the default value for an integer is 0, and the default value for a string is null.
Here is an example of declaring an array with default values:
boolean[] flags = new boolean[4];
This code creates a boolean array named flags
with four elements and assigns them the default value of false.
Method 5: Using the Arrays Class
The Arrays class is a built-in Java class that provides several methods that simplify array manipulation. One of these methods is the asList()
method, which allows you to convert an array to a list.
Here is an example of using the Arrays class:
String[] myWords = {"Hello", "World"};
List<String> myList = Arrays.asList(myWords);
This code creates a String array named myWords
with two elements and converts it to a list using the Arrays.asList()
method.
Benefits and Drawbacks of Each Method
Each method has its benefits and drawbacks. For example, the “new” keyword method is flexible and useful when you don’t know the values to be stored in the array at the time of declaration, but it can be slower than other methods.
On the other hand, the shorthand syntax is concise and easy to read, but it cannot be used when you require more complex array initialization.
How to Initialize an Array in Java
Initializing an array involves assigning a value to each element in the array. There are different ways to initialize an array in Java.
Common Mistakes Made by Beginners
One common mistake made by beginners is not initializing all the elements of an array. If you fail to do so, Java automatically assigns default values to the uninitialized elements. Another common mistake is using an invalid index value when assigning values to array elements.
Different Ways to Declare an Array with Initialization
Here are some methods for initializing an array in Java:
String[] myWords = {"Hello", "World"};
This code initializes a String array named myWords
with two elements.
int[] numbers = new int[]{1, 2, 3};
This code initializes an integer array named numbers
with three elements.
char[] vowels = {'a', 'e', 'i', 'o', 'u'};
This code initializes a character array named vowels
with five elements.
Code Examples for Each Method
Here is an example of initializing an array using the shorthand syntax:
int[] numbers = {1, 2, 3, 4, 5};
This code initializes an integer array named numbers
with five elements and assigns them the values 1, 2, 3, 4, and 5.
Practical Tips for Efficient Array Declaration in Java
Efficient array declaration is essential for optimizing performance in Java programs. Here are some tips for efficient array declaration in Java:
- Always declare the array with an appropriate size to avoid unnecessary resizing operations.
- Consider using primitive arrays instead of object arrays for performance improvement.
- Use the enhanced for loop for iterating through array elements whenever possible for better code readability and performance.
Explanation and Code Examples for Each Technique
Here is an example of using the enhanced for loop for iterating through array elements:
for (int number : numbers) {
System.out.println(number);
}
This code uses the enhanced for loop to iterate through each element in the integer array named numbers
and print out its value.
Comparing Different Approaches to Declare Arrays in Java
Different approaches to declaring arrays have their benefits and drawbacks. Here is a comparison of the different methods:
Method | Benefits | Drawbacks |
New Keyword | Flexible | Slower |
Short-Hand Syntax | Concise and easy to read | Cannot be used for more complex initialization |
Anonymous Array | Useful for temporary arrays | Cannot be reused, so not suitable for permanent arrays |
Default Values | Useful for assigning default values to array elements | Not suitable for more complex initialization |
Arrays Class | Provides many useful methods to simplify array manipulation | Can be slower than other methods |
Suggestions on When to Use Each Approach Based on Use Cases
Choose the appropriate method for array declaration based on the use case. For example, if you need flexibility in array initialization, use the “new” keyword method. If you require concise and easy to read code, use the shorthand syntax method. If you need a temporary array, use the anonymous array method, and if you need to assign default values to array elements, use the default values method.
Conclusion
Declaring arrays correctly in Java is essential for efficient programming and optimal performance. This article provided a comprehensive guide on how to declare an array in Java, covering different methods, techniques, and best practices. Remember to choose the appropriate method based on the use case and always initialize all array elements to avoid common beginner mistakes.