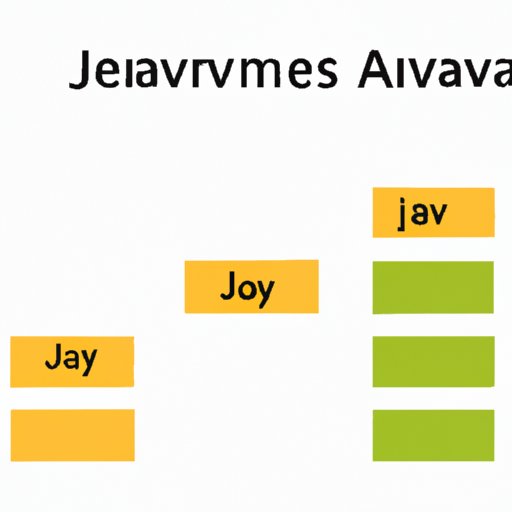
Introduction
If you’re interested in Java programming, understanding how to create arrays in Java is a crucial skill to have in your arsenal. Arrays are data structures that allow you to store and manipulate multiple values in a single object, making them essential for many programming applications. In this article, we’ll take a comprehensive look at how to create and manipulate arrays in Java, including different types of arrays, tips for working with arrays, common mistakes to avoid, and real-world applications.
A Step-by-Step Guide to Creating Arrays in Java
Arrays are a fundamental part of Java programming. At their most basic level, arrays are used to store collections of data in a single object. You can create an array with a single command in Java:
“`java
dataType[] arrayName = new dataType[arraySize];
“`
Here, `dataType` refers to the data type of the elements you want to store in the array, `arrayName` is the name you give to the array, and `arraySize` is the number of elements you want to store in the array.
For example, to create an array of integers called `myArray` that holds 10 elements, you would write:
“`java
int[] myArray = new int[10];
“`
Once you’ve created an array in Java, you can assign values to it with a simple loop:
“`java
for(int i=0; i
Beginners to Java programming often make mistakes when creating arrays, particularly with respect to array indices. One common error is forgetting that array indices begin at 0, not 1. Another common error is trying to access an element of the array that does not exist, which can result in an “array out of bounds” error.
The Different Types of Arrays in Java
Java offers a variety of array types, each with its own unique characteristics and use cases. Here are a few of the most common:
One-Dimensional Arrays
One-dimensional arrays are the simplest type of array; with a single index, they allow you to store a list of values in a single object. One-dimensional arrays can be populated with values either during initialization or by assigning values to each element of the array individually.
Two-Dimensional Arrays
Two-dimensional arrays offer a more complex version of one-dimensional arrays. They can be thought of as an “array of arrays,” with each element of the array itself being another array. Two-dimensional arrays are often used to represent tables of data.
Code Examples: Creating and Manipulating Arrays
Here’s an example of how you could create a one-dimensional array of integers:
“`java
int[] myArray = {2,4,6,8,10};
“`
Here’s an example of how you could create a two-dimensional array of strings:
“`java
String[][] myArray = {
{“row 1, column 1”, “row 1, column 2”},
{“row 2, column 1”, “row 2, column 2”},
{“row 3, column 1”, “row 3, column 2”}
};
“`
Once you’ve created an array, there are a number of ways to manipulate it. For example, you can use the `Arrays.sort()` method to sort an array in ascending order:
“`java
Arrays.sort(myArray);
“`
Tips for Working with Arrays in Java
Arrays can be complex to work with, particularly as the number of elements in the array grows. Here are a few tips for optimizing your use of Java arrays:
Sorting and Filtering Arrays
One common technique for working with arrays is filtering them based on particular criteria. For example, you might want to sort an array based on the values of particular elements; to do so, you could use the `Arrays.sort()` method.
Optimizing Array Performance
As with any code, optimizing array performance is crucial to the success of your program. One approach to optimizing array performance is to initialize the array to its maximum possible size when creating it. This can help prevent the need to resize the array later, which can be a computationally expensive process.
Real-World Applications of Arrays in Java
Arrays have a wide variety of use cases in Java programming. For example, they might be used to store and sort employee data in a company-wide database, or to hold real-time stock price data in a financial application. Whatever the application, arrays are critical tools for managing and manipulating large sets of data.
Common Mistakes to Avoid When Creating Arrays in Java
Creating Java arrays can be tricky, particularly for beginners. Here are a few common mistakes to avoid when working with arrays:
Forgetting to Initialize the Array
One of the most common errors when creating Java arrays is forgetting to initialize the array with the `new` keyword. Doing so will result in a “null pointer exception” error when you try to access the array later.
Off-By-One Errors
Another common error is making off-by-one errors when accessing elements of the array. This occurs when the programmer forgets that array indices start at 0, not 1. Such mistakes can lead to hard-to-detect bugs in the code.
The Syntax of Java Arrays
Finally, let’s take a deep dive into the technical syntax of Java arrays. Here are a few key commands and syntax rules to keep in mind when working with Java arrays:
Declaration
To declare an array in Java, you use the following syntax:
“`java
dataType[] arrayName;
“`
Here, `dataType` refers to the type of the elements that the array will hold.
Initialization
To initialize an array in Java, you use the following syntax:
“`java
arrayName = new dataType[arraySize];
“`
Here, `arraySize` refers to the number of elements that the array will hold.
Accessing Elements of an Array
To access the elements of an array in Java, you use the following syntax:
“`java
arrayName[index];
“`
Here, `index` refers to the index of the element you want to access.
How to Use Arrays in Real-World Java Applications
Arrays have a wide variety of use cases in real-world Java applications. For example, they might be used to store and sort employee data in a company-wide database, or to hold real-time stock price data in a financial application. Whatever the application, arrays are critical tools for managing and manipulating large sets of data.
Examples of Real-World Array-Based Solutions
Here are a few examples of real-world Java applications in which arrays are commonly used:
Sorting Algorithms
One of the most important real-world applications of Java arrays is in sorting algorithms. These algorithms are used in a wide variety of applications, from processing large datasets to sorting search results.
Data Structures
Arrays are also used in a variety of other data structures, including linked lists, stacks, and queues. By using arrays in these data structures, programmers can manage and manipulate data in new and more efficient ways.
Graphical User Interfaces (GUIs)
Finally, arrays are often used in graphical user interfaces (GUIs). By using arrays to store GUI elements, programmers can create rich graphical interfaces that are more intuitive and user-friendly.
Conclusion
In this article, we’ve explored the ins and outs of creating and manipulating arrays in Java. From creating basic one-dimensional arrays to optimizing their performance and using them in real-world applications, we’ve covered everything you need to know to get started with arrays in Java programming. Remember to take the time to learn the syntax of arrays and avoid common mistakes to ensure that your code is clean, functional, and efficient. By mastering arrays in Java, you’ll be well on your way to becoming a skilled and successful Java programmer.