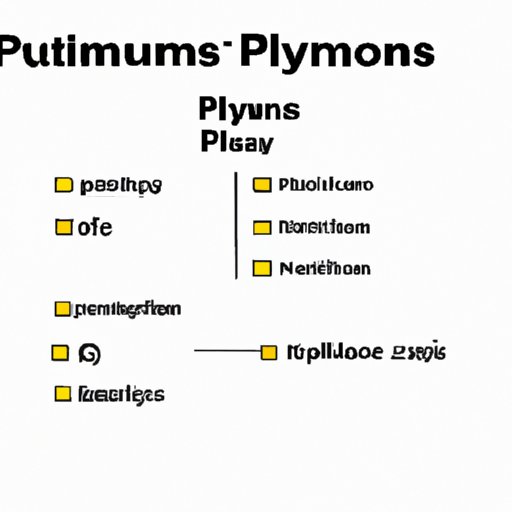
I. Introduction
Python functions are an essential part of programming as they are reusable blocks of code designed to perform specific tasks. Calling functions in Python is crucial as it allows you to utilize the code you have written, which saves time and effort in the long run.
This article will provide a comprehensive guide on how to call a function in Python. We will cover the different methods of calling functions and how to define arguments. We will also provide real-world examples, common issues and troubleshooting tips, as well as additional resources for further learning.
II. Creating a Function
Before we dive into calling a function in Python, let’s define what a function is. A function is a block of code that performs a specific task. You can use it multiple times in your code without having to rewrite the same lines over and over again.
The syntax for defining a function in Python is straightforward. You use the “def” keyword, followed by the name of your function, and parentheses that may or may not contain parameters. Here’s an example:
“`
def my_function():
print(“Hello, World!”)
“`
The above code block defines a function named “my_function” that prints “Hello, World!” when called. Notice the colon at the end of the first line. This is required to define the beginning of a new block of code. The code inside the parentheses will be executed when the function is called.
Function parameters are values that are passed to a function when it’s called. They allow you to pass input data to your function and modify its behavior accordingly. Let’s see an example:
“`
def greet(name):
print(“Hello, ” + name)
“`
The above code block defines a function named “greet” that takes in a parameter called “name.” When the function is called, it will print “Hello, ” followed by the name passed to it.
III. Defining Arguments
Arguments or parameters are values that are passed to a function when it’s called. They allow you to pass input data to your function and modify its behavior accordingly. There are three types of arguments in Python:
- Positional arguments: These arguments are passed to a function in the order that they appear. For example:
- Default arguments: Default arguments are used when a value is not specified for the parameter. For example:
- Keyword arguments: With keyword arguments, you can specify the name of the argument when you pass the value. This makes it easier to keep track of the arguments. For example:
“`
def greet(name, age):
print(“Hello, ” + name + “!”)
print(“You are ” + age + ” years old.”)
greet(“John”, “25”)
“`
“`
def greet(name = “User”, age = “18”):
print(“Hello, ” + name + “!”)
print(“You are ” + age + ” years old.”)
greet()
“`
“`
def greet(name, age):
print(“Hello, ” + name + “!”)
print(“You are ” + age + ” years old.”)
greet(age = “25”, name = “John”)
“`
IV. Calling a Function Using Different Methods
Now that we have covered defining arguments, let’s dive into the different methods of calling a function:
Method 1: Call function using parameters and positional arguments
This method is useful when you know the number of parameters your function accepts and their order. Here’s an example:
“`
def add_numbers(num1, num2):
print(num1 + num2)
add_numbers(10, 20)
“`
The above code block defines a function named “add_numbers” that takes two parameters called “num1” and “num2.” When the function is called with two arguments (10 and 20), it will print their sum.
Method 2: Call function using default arguments
This method is useful when you want to provide default values for your function parameters. If an argument is not passed, it will use the default value instead. Here’s an example:
“`
def greet(name = “User”, age = “18”):
print(“Hello, ” + name + “!”)
print(“You are ” + age + ” years old.”)
greet()
“`
The above code block defines a function named “greet” that takes two parameters called “name” and “age.” However, we have provided default values for these parameters. When the function is called without any arguments, it uses the default values instead.
Method 3: Call function using keyword arguments
This method is useful when you want to specify the name of the argument when you pass the value. It makes it easier to keep track of the arguments. Here’s an example:
“`
def greet(name, age):
print(“Hello, ” + name + “!”)
print(“You are ” + age + ” years old.”)
greet(age = “25”, name = “John”)
“`
The above code block defines a function named “greet” that takes two parameters called “name” and “age.” When the function is called, we have specified the name of each argument. This makes it easier to keep track of what’s being passed to the function.
Method 4: Call function using unpacking arguments
This method is useful when you want to pass a list or a tuple as arguments to a function. You can use the “*” operator to unpack the sequence and pass each element as a separate argument. Here’s an example:
“`
def add_numbers(num1, num2):
print(num1 + num2)
numbers = [10, 20]
add_numbers(*numbers)
“`
The above code block defines a function named “add_numbers” that takes two parameters called “num1” and “num2.” We have created a list called “numbers” with two elements. By using the “*” operator to unpack the list, we are passing each element as a separate argument to the function.
V. Examples to Illustrate Different Ways to Call a Function
Let’s take a look at some real-world examples to demonstrate calling Python functions:
Example 1: Calculating the Area of a Circle
Here’s an example of a function that calculates the area of a circle:
“`
import math
def area_circle(radius):
area = math.pi * (radius ** 2)
return area
print(area_circle(10))
“`
The above code block defines a function called “area_circle” that takes a parameter called “radius.” It then calculates the area of a circle using the formula (pi * radius^2) and returns the result. The function is called with an argument of 10, and the result is printed to the console.
Example 2: Checking if a Number is Prime
Here’s an example of a function that checks if a number is prime:
“`
def is_prime(number):
if number > 1:
for i in range(2, number):
if number % i == 0:
print(number, “is not prime”)
break
else:
print(number, “is prime”)
else:
print(number, “is not prime”)
is_prime(17)
“`
The above code block defines a function called “is_prime” that takes a parameter called “number.” It then checks if the number is prime by iterating through each number between 2 and the number itself. If it finds a number that divides it without a remainder, it means the number is not prime. Otherwise, it’s prime. The function is called with an argument of 17, and it prints “17 is prime” to the console.
Example 3: Sorting a List of Tuples
Here’s an example of a function that sorts a list of tuples based on the second item in each tuple:
“`
def sort_tuples(data):
data.sort(key = lambda x: x[1])
return data
print(sort_tuples([(1, 5), (2, 3), (3, 4)]))
“`
The above code block defines a function called “sort_tuples” that takes a parameter called “data.” It then sorts the list of tuples based on the second item in each tuple and returns the sorted list. The function is called with an argument containing three tuples, and the result is printed to the console.
Comparison of Different Methods to Call Functions
Now that we have seen some examples of calling Python functions, let’s compare the different methods:
- Using positional arguments is the most basic way of calling a function. It’s useful when you know the number of arguments your function accepts and their order.
- Using default arguments is useful when you want to provide default values for your function parameters. If an argument is not passed, it will use the default value instead.
- Using keyword arguments is useful when you want to specify the name of the argument when you pass the value. This makes it easier to keep track of the arguments.
- Using unpacking arguments is useful when you want to pass a list or a tuple as arguments to a function. You can use the “*” operator to unpack the sequence and pass each element as a separate argument.
Pros and Cons of Each Approach
Each approach has its pros and cons. Using positional arguments is simple and straightforward, but it can be confusing if you have a lot of arguments in your function. Using default arguments makes it easier to set default values, but it can make it difficult to understand what the function does if there are too many default arguments. Using keyword arguments is useful for readability, but it can make the code longer. Using unpacking arguments makes it easier to pass a sequence of elements but can be confusing if the sequence has too many elements.
VI. Q&A: Answering Common Questions About Calling Functions in Python
Q1. Can a function return more than one value?
Yes, a function can return more than one value using tuples. Here’s an example:
“`
def get_name_and_age():
name = “John”
age = 25
return name, age
name, age = get_name_and_age()
print(name, age)
“`
The above code block defines a function called “get_name_and_age” that sets the name and age variables and returns them as a tuple. The function is then called, and the returned tuple is unpacked into the “name” and “age” variables.
Q2. What happens if you call a function with the wrong number of arguments?
If you call a function with the wrong number of arguments, you will get a TypeError. For example:
“`
def add_numbers(num1, num2):
print(num1 + num2)
add_numbers(10)
“`
The above code block defines a function called “add_numbers” that takes two parameters called “num1” and “num2.” When the function is called with only one argument, you will get a TypeError.
Q3. Can you call a function within another function?
Yes, you can call a function within another function. Here’s an example:
“`
def greet(name):
print(“Hello, ” + name + “!”)
def greet_user():
name = input(“What’s your name?”)
greet(name)
greet_user()
“`
The above code block defines two functions. The first function called “greet” takes a parameter called “name” and prints a greeting. The second function called “greet_user” asks for the user’s name and calls the “greet” function with the name parameter.
VII. Conclusion
In conclusion, calling functions in Python is an essential part of programming. It allows you to reuse your code, making it easier to maintain and modify. In this article, we covered the different methods of calling functions, including using parameters and positional arguments, default arguments, keyword arguments, and unpacking arguments.
We also provided real-world examples to demonstrate calling Python functions, compared the different methods, and discussed the pros and cons of each approach. Finally, we answered common questions about calling functions in Python.
We encourage readers to practice what they have learned and explore further resources for learning Python functions.